【TypeScript】Expressとmongooseで開発環境構築
公開
更新
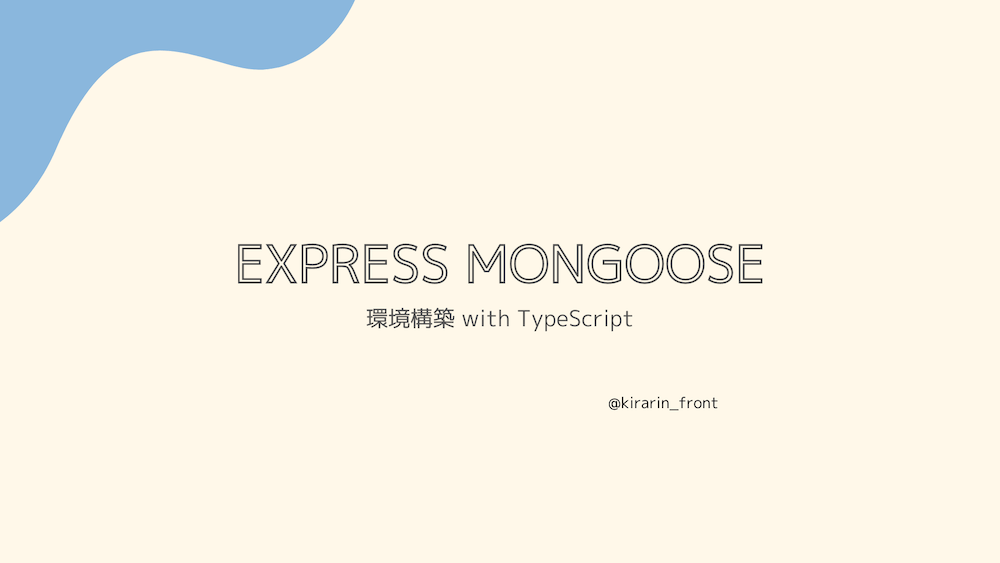
前提
MongoDBとNode.jsはインストール前提で進めます。
すでにJavaScriptでその環境で開発したことある方向けです。
プロジェクトの作成
好きな名前でディレクトリ名を作成。
↓
作成したディレクトリに移動。
↓
package.json作成。
オプションに -y をつけることで問答を飛ばすことができる。
mkdir dir-name
cd dir-name
npm init -y // -yで問答飛ばし
パッケージインストール
dependenciesとdevDependenciesの違い
dependencies・・・ビルドファイルに含まれるパッケージ。つまり本番環境でも使用されるパッケージ。
devDependencies・・・ビルドファイルに含まれないパッケージ。開発環境でのみ使用するパッケージ。(例:TypeScript)
基本的にオプションをつけなければdependenciesに入って、
npmなら-DオプションでdevDependenciesに入る。
インストール
express
とmongoose
をインストール。
※今回view側の設定・解説は省きます。
npm i express mongoose
TypeScript
とtypes
パッケージをインストール
この辺のパッケージは本番環境のビルドファイルに含める必要がないので、
-Dオプションをつけてインストール。
typesファイル・・・パッケージの型定義ファイル
ts-node-dev・・・TypeScript
をコンパイルせずにローカルサーバーを立ち上げることができる。
ts-node と ts-node-dev の違い
npm i -D typescript ts-node-dev @types/node @types/express @types/mongoose
express設定
メインファイルを作成
mkdir src
cd src
touch index.ts
express
の初期設定
// index.ts
import express, { Request, Response } from 'express'
const app = express()
// req.bodyをパース
app.use(express.urlencoded({ extended: true }))
app.get('/', (req: Request, res: Response) => {
res.send('成功!')
})
// localhost://3000でローカルサーバーを起動
app.listen(3000, () => {
console.log('ポート3000で待ち受け中...')
})
mongoose設定
ディレクトリ/ファイル
mkdir models
cd models
touch pokemon.ts
スキーマとモデルの作成
import { Schema, model } from 'mongoose'
interface Pokemon {
name: string
booksId: number
}
const pokemonSchema = new Schema<Pokemon>({
name: {
type: String,
required: true,
},
bookId: {
type: Number,
required: true,
min: 1,
max: 898
}
})
export const Pokemon = model<Pokemon>('Pokemon', pokemonSchema)
MongoDBサーバーに接続
// index.ts
import mongoose from 'mongoose'
import { Pokemon } from './models/pokemon'
mongoose
// pokemonLandという名前でDBが作成される。
.connect('mongodb://localhost:27017/pokemonLand', {
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(() => {
console.log('MongoDBコネクションOK!!')
})
.catch((err) => {
console.log('MongoDBコネクションエラー')
console.log(err)
})
サーバーの設定
package.json
にscript
追加
"scripts": {
"start": "ts-node-dev src/index.ts"
},
ts-node-dev
でsrc/index.ts
を読み込んでサーバーを起動する。
サーバー起動
ts-node-devが起動してサーバーが立ち上がる。
npm start
localhost:3000/
にアクセスして「成功!」と表示されればセットアップ完了!